Index Of /
Dart: calculate the dates difference in years, months and days
Otober 20, 2019
I'm playing with Flutter in my free time for a week, trying to build a simple app with my kids' measurements (height, weight, etc).
One of the first things I wanted to show is their age. Not just years, but also months and days, because they are constantly asking me about it: exactly how old are they. So I decided to show this in the interface.
I was pretty excited when I saw that there is a special method in Dart about calculating the date differences: DateTime.difference
but unfortunately, only the difference measured in days is supported (issue #1426).
But I've already wanted this feature, so remembering JavaScript way to achieve this, I created my method, returning the years, months and days as a single object. Here it is:
class KidsAge {
int years;
int months;
int days;
KidsAge({ this.years = 0, this.months = 0, this.days = 0 });
}
KidsAge getTheKidsAge(String birthday) {
if (birthday != '') {
var birthDate = DateTime.tryParse(birthday);
if (birthDate != null) {
final now = new DateTime.now();
int years = now.year - birthDate.year;
int months = now.month - birthDate.month;
int days = now.day - birthDate.day;
if (months < 0 || (months == 0 && days < 0)) {
years--;
months += (days < 0 ? 11 : 12);
}
if (days < 0) {
final monthAgo = new DateTime(now.year, now.month - 1, birthDate.day);
days = now.difference(monthAgo).inDays + 1;
}
return KidsAge(years: years, months: months, days: days);
} else {
print('getTheKidsAge: not a valid date');
}
} else {
print('getTheKidsAge: date is empty');
}
return KidsAge();
}
The logic here is as follows:
- First, checking is the date valid. In case if it is not — returning an empty object.
- Next — getting initial values. Subtract the asked years, months and days from the current date.
- Then in case if the months' difference is negative or if it's zero and days diff is negative — the date has not yet arrived this year. So we are subtracting the one year and adding 12 (or 11, in case of negative days' diff) to the months' diff.
- The last step — in case of negative days' diff have to add the length of the previous month... but I wanted to play a bit with the difference method and get the difference in days between the today and the day from the date if it was last month.
That's it for today. I hope that will help somebody.
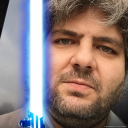
Some frontender thoughts
Twitter,
GitHub,
LinkedIn